In this post, we will learn how to add TypeScript to NodeJS. I will guide you step by step through the whole process, so let’s start!
For the video version, check it out below
What is TypeScript?
TypeScript is a superset of JavaScript that adds static typing and other modern features to the language. It is developed and maintained by Microsoft and is widely used for building large-scale, maintainable applications.
TypeScript compiles to plain JavaScript, so it can run anywhere JavaScript runs, including browsers and Node.js.
The official TypeScript documentation can be found here: https://www.typescriptlang.org/docs
How to add Typescript to NodeJS app – What do we need?
For this tutorial, we need to create a very very basic simple app with NodeJS and Express, so let’s go ahead and do just that!
First, create a folder. I’ve named mine server, you can name your folder as you like. Now cd into the folder and run the following command:
npm init -y
The npm init command can be used to set up a new or existing npm package.
Also run the following command to install TypeScript and its supporting tools:
npm install typescript ts-node @types/node express @types/express --save-dev
typescript
: TypeScript compiler.ts-node
: Allows you to run TypeScript directly in Node.js without pre-compiling.@types/node
: Type definitions for Node.js.@types/express
: Type definitions for Express.- express: A fast, unopinionated, minimalist web framework for Node.js, providing a robust set of features for web and mobile applications.
Then, create a src folder and inside of it, an index.ts file, that will hold all our application’s code:
import express, { Request, Response } from "express";
const app = express();
const PORT = process.env.PORT || 3000;
// Middleware
app.use(express.json());
// Routes
app.get("/", (req: Request, res: Response) => {
res.send("Hello, TypeScript with Express!");
});
// Start the server
app.listen(PORT, () => {
console.log(`Server is running on http://localhost:${PORT}`);
});
This code creates a simple web server using Express.js and TypeScript. It listens for HTTP requests on a specified port (defaulting to 3000) and responds with the message "Hello, TypeScript with Express!"
when a GET
request is made to the root URL (/
). It also uses middleware to parse incoming JSON data in the request body.
Now that our server is in place, lets run the following command:
npx tsc --init
This creates a default tsconfig.json
. Update it for Node.js and Express:
{
"compilerOptions": {
"target": "ES2020",
"module": "commonjs",
"rootDir": "./src",
"outDir": "./dist",
"strict": true,
"esModuleInterop": true,
"skipLibCheck": true
},
"include": ["src/**/*"],
"exclude": ["node_modules"]
}
Add a compile and a start script in package.json
The compile
script is a separate step to explicitly compile TypeScript into JavaScript before running it. This is useful in production or staging environments where you want to pre-compile your code for faster startup times. The start
script then runs the compiled JavaScript code, ensuring that the server is using the JavaScript output instead of relying on ts-node
to run the TypeScript files directly.
TypeScript requires compilation to JavaScript before it can be executed in Node.js. In a development environment, ts-node
allows you to run TypeScript directly without compiling first, but in production, you often want to compile the TypeScript files ahead of time.
To create a compile script and a start script in your package.json
, follow these steps:
In the package.json file, under the scripts key, add these lines:
"compile": "tsc",
"start": "npm run compile && node ./dist/index.js"
Your file should look like this:
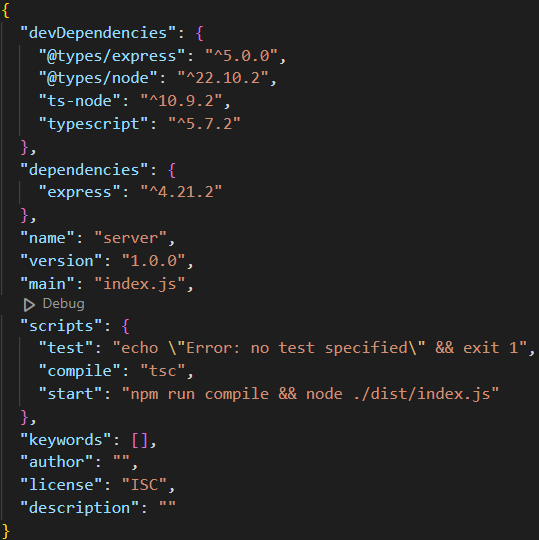
As a best practice, you should NOT commit your dist folder to git. To do that, create a .gitignore file under the server directory, at the same level with your package.json, with the following content:
node_modules/
build/
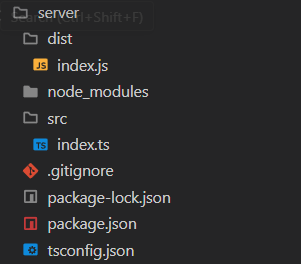
Now, to start our server and check if everything is working, we should cd in the server folder(if u already didn’t) and run the command that we wrote earlier:
npm run start
You should get an output similar to this:
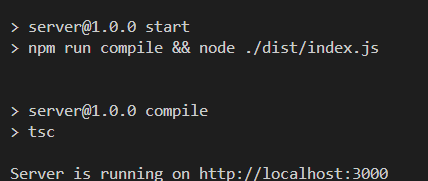
If you open up your browser on http://localhost:3000/ , we should see our app running:
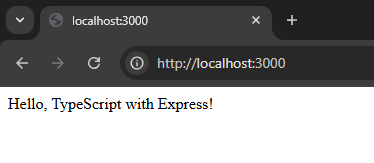
Congratulations, you just found out how to add TypeScript to NodeJS for your application!
If you enjoy the content, please leave a comment below and also, maybe you can check this awesome post if you’re interested into DevOps: https://developercuisine.net/best-kubernetes-books/
Recent Comments