If you’re working with React JS, understanding how to use the useEffect
hook is essential for handling side effects in functional components. Whether you’re fetching data, setting up subscriptions, or manipulating the DOM, useEffect
in React JS is your go-to tool. In this guide, we’ll break down everything you need to know about useEffect
—from basic usage to best practices and common pitfalls.
Official documentation for useEffect in React JS: https://react.dev/reference/react/useEffect
What is useEffect
in React JS?
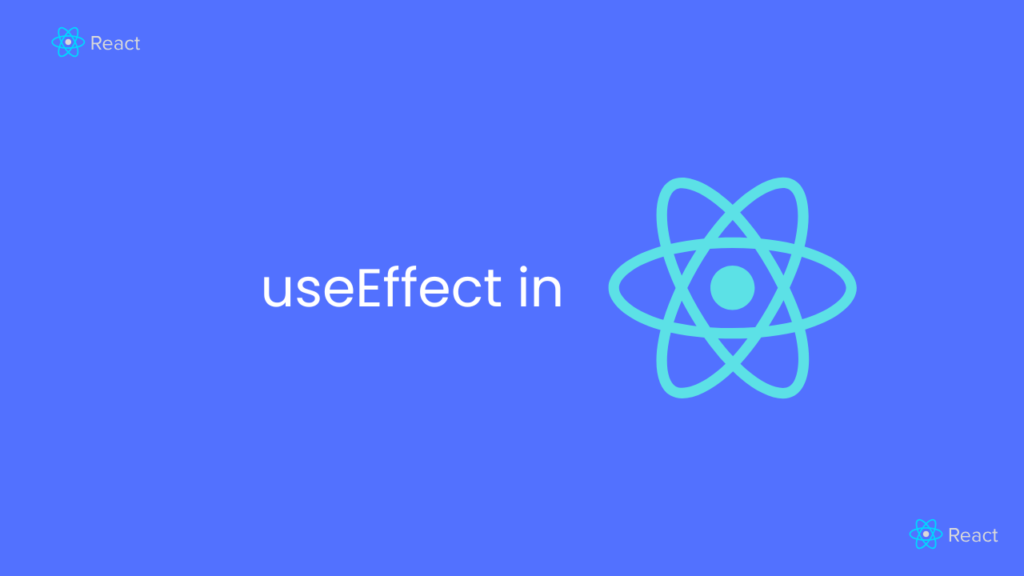
In React JS, useEffect
is a hook introduced in React 16.8 that allows you to perform side effects in function components. Side effects include things like:
- Fetching data from an API.
- Manipulating the DOM.
- Setting up subscriptions or timers.
Here’s a simple example:
import React, { useState, useEffect } from 'react';
function Example() {
const [count, setCount] = useState(0);
useEffect(() => {
console.log(`You clicked ${count} times`);
});
return (
<div>
<p>You clicked {count} times</p>
<button onClick={() => setCount(count + 1)}>Click me</button>
</div>
);
}
How Does useEffect
Work?
The useEffect
hook takes two arguments:
- A callback function: This function contains the side effect logic.
- An optional dependency array: Controls when the effect runs.
Key Concepts of useEffect
1. Running After Every Render
By default, useEffect
runs after every render.
useEffect(() => {
console.log('Effect ran');
});
2. Using the Dependency Array
The dependency array is a list of values that useEffect
watches. The effect runs only when one of these values changes.
Example:
useEffect(() => {
console.log(`Count is ${count}`);
}, [count]);
- Empty Dependency Array:
useEffect
runs only once, similar tocomponentDidMount
.
useEffect(() => {
console.log('Effect ran only once');
}, []);
- No Dependency Array:
useEffect
runs after every render.
3. Cleaning Up Effects
Some effects, like subscriptions or timers, require cleanup. useEffect
allows you to return a cleanup function.
Example:
useEffect(() => {
const timer = setInterval(() => {
console.log('Timer running');
}, 1000);
return () => {
clearInterval(timer); // Cleanup on unmount
};
}, []);
Common Use Cases for useEffect
1. Fetching Data
useEffect(() => {
async function fetchData() {
const response = await fetch('https://api.example.com/data');
const data = await response.json();
console.log(data);
}
fetchData();
}, []);
2. Subscribing to Events
useEffect(() => {
const handleResize = () => console.log(window.innerWidth);
window.addEventListener('resize', handleResize);
return () => window.removeEventListener('resize', handleResize);
}, []);
3. Animating Components
useEffect(() => {
const element = document.querySelector('.my-div');
element.style.transition = 'opacity 0.5s';
element.style.opacity = 1;
return () => {
element.style.opacity = 0; // Reset on unmount
};
}, []);
Best Practices for useEffect
in React JS
- Keep Effects Focused
EachuseEffect
should handle one concern to maintain readability and prevent bugs. - Avoid Overusing Effects
Sometimes effects are unnecessary. For instance, derived state can often replace an effect. - Manage Dependencies Carefully
Always include all external variables used in the effect in the dependency array. - Handle Cleanup
Ensure your effects clean up to avoid memory leaks.
Common Pitfalls of useEffect
in React JS
1. Infinite Loops
Incorrectly setting dependencies can lead to infinite re-renders.
useEffect(() => {
setCount(count + 1); // This will cause an infinite loop
}, [count]);
Solution: Avoid updating state directly inside the effect unless it’s wrapped in a condition or logic.
If you liked this post make sure to check out our previous post too: https://developercuisine.net/html-div/
Recent Comments